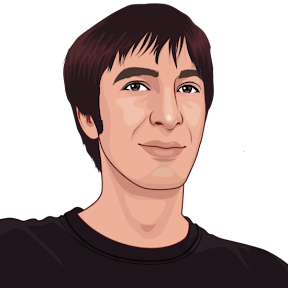
Web developer & teacher @saaslit
How to iterate over a Ruby array
A short memo about how to iterate over a Ruby array
The classic way to iterate over a Ruby array
Let's take a simple Ruby array :
a = [11, 'foo', 42]
You can use the .each
method
a.each { |x| puts x }
# 11
# foo
# 42
If you are confused about the syntax, I wrote an article about Ruby blocks, procs and lambda, so it's equivalent to the following :
a.each do |x|
puts x
end
# 11
# foo
# 42
The for loop
The for loop is available in Ruby, to be honest I don't see it very often, so I put it here as reference :
for e in a
puts e
end
# 11
# foo
# 42
each_with_index
for a Ruby array
If you need to know about the index, the following syntax is available :
a.each_with_index {|e, index| puts "#{e} => #{index}" }
# 11 => 0
# foo => 1
# 42 => 2
Again, this is equivalent to :
a.each_with_index do |e, index|
puts "#{e} => #{index}"
end
# 11 => 0
# foo => 1
# 42 => 2
Do you want to create an array from an existing one?
You probably want the .map
method here
a.map {|e| e.to_s + ' z' }
# ["11 z", "foo z", "42 z"]
You actually iterate on the array, in order to extract an array of the same size.
Note that array "a" is left unchanged.
Lastly, I wrote an article about how to map with index in Ruby.
Do you want to reject unwanted values?
Now it's .reject
a.reject {|e| e.is_a?(String) }
# [11, 42]
a.reject {|e| e.is_a?(Numeric) }
# ["foo"]
Conclusion
Iterate over a Ruby array is quite simple, given it's a big classic in programming. Just take time to feel at ease with the block notation, it's more or less like the anonymous JS functions. If you have a specific need, also have a look at the Enumerable module.